Go - Getting Started
It's finally time for my very first programming article. In the past, you have seen articles about Ansible, Podman and Linux, mostly. But what about making your own program? In this article, we will get started with Go.
It's finally time for my very first programming article. In the past, you have seen articles about Ansible, Podman and Linux, mostly. But what about making your own program? In this article, we will get started with Go.
Let's install, code and compile, shall we?
Go(lang)
Go or Golang is a statically typed, compiled, high level programming language designed at Google. It aims to improve programming productivity for network machines, multicore applications and large code bases.
To make the life of a programmer easy, it comes with memory safety, garbage collection, structural typing and CSP-style concurrency. In addition, it is considered to produce reliable code, which should be easy to maintain and use.
Well, and it compiles to a single binary, has an awesome ecosystem and documentation. Oh, and it has an awesome, cute mascot – a gopher.
But is Go really used for production software? Well, it is. You can find a lot of Go in Kubernetes, Gitea, Podman or etcd.
Install
Installing Go is pretty easy and included all major Linux distributions. With just a single command, you should be good to Go.
# Install go in Fedora, CentOS, AlmaLinux
$ sudo dnf install go
But, you will be bound to the supported version of Go, when using the distribution packages. This is not an issue if you start with Go, as long as you stick to tutorials that match your version of Go. In case you want to use another version or even multiple versions of Go, there is another, officially supported way.
# Ensure, that you don't have go installed
$ sudo dnf remove go
# Remove old go installations
$ sudo rm -rf /usr/local/go
# Download a recent version
$ wget https://go.dev/dl/go1.21.3.linux-amd64.tar.gz
# Unpack the archive
$ sudo tar -C /usr/local -xzf go1.21.3.linux-amd64.tar.gz
Be aware, that the downloaded version must match your architecture and operating system. You can find all downloads at the official download page. By the way, updating works exactly the same way.
There is one more thing, you need to do. /usr/local/go
is not known by your system right now. Therefore, you need to add a single line at the end of your .bashrc
file or another location to update your path.
...SNIP...
# Add gopath to PATH
export PATH=$PATH:/usr/local/go/bin
~/.bashrc
Finally, you can test, if Go works.
# Check go version
$ go version
go version go1.21.3 linux/amd64
# Check go location
$ which go
/usr/local/go/bin/go
In case you want to install another version, this can be done according to the guide.
Hello World!
Now that you have Go installed, it's time for the almighty, well known Hello, World!
project. Create a new directory and you are ready to start.
Initialize the project
To make Go aware, that the directory is meant to be a Go folder, you need to run a single command.
# Initialize Go
$ go mod init <name_of_project>
Go recommends naming the project like the repository where it lives. Therefore, I am using the below command.
# Initialize my project
$ go mod init github.com/dschier-wtd/go-hello-world
This command will create a new file go.mod
. The content is pretty minimal, right now, but will grow the more your project grows.
module github.com/dschier-wtd/go-hello-world
go 1.21.3
go.mod
The source file(s)
Next, you will need something to write your code in. For the start, you can use a simple main.go
file. There are more sophisticated approaches for larger projects, but a single file is fine for now. Anyway, it is recommended to put it in a proper structure right away.
$ tree
.
├── cmd
│ └── hello
│ └── main.go
└── go.mod
3 directories, 2 files
This follows the common Go standard. You should read about it, before digging into larger projects. Anyway, let's finally write some code.
A hello-world
application is super easy to create. We just require some simple lines of code. Let me show you an example and comment it for better understanding.
package main // The name of your package
import ( // Import other packages and modules
"fmt" // Import the fmt package
)
func main() { // The main function, that will always run
fmt.Println("Hello, World!") // Using the fmt package to print
}
We are using the fmt
package from the Go standard packages. It allows to print and format various outputs. This also one of the major concepts of Go. Other than JAVA or C++, Go works with functions. Keeping these functions small and well-ordered/sorted, makes it pretty easy to read Go code.
Run Go Run
Now, we want to run our code, tight? Go comes with some functionality that makes it easy to run, test and compile our code.
# Test run your code
$ go run cmd/hello/main.go
Hello, World!
# Compile it to a binary
$ go build cmd/hello/main.go
# Execute the binary
$ ./main
Hello, World!
And that's already it (for now). We do have the most simple Go application.
Where to go from here?
Learning a new programming language can feel awesome or frustrating. Often both feelings are present and mix with each other. Just be aware, that everybody started with something new at some point in time. Nobody was born as the Go programmer, Python developer or DevOps specialist.
For me, it is important to have a concrete project to work on. Usually, I am looking for tasks that require more work and that can be learned. If I want to have some nice herbs and fresh chilies, I start with gardening. With Go, it was about a small, but fast screen fetch alternative. You can find it in the link below.

To get you started, you might want to check out some courses and tutorials that I found helpful and interesting.

Docs & Links
Below, you can find a couple of links that might help you with the first steps or finding inspiration for your first project.
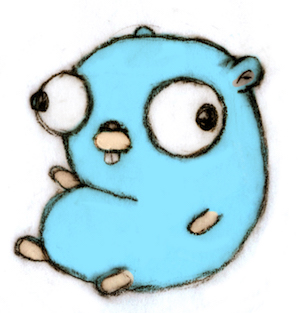
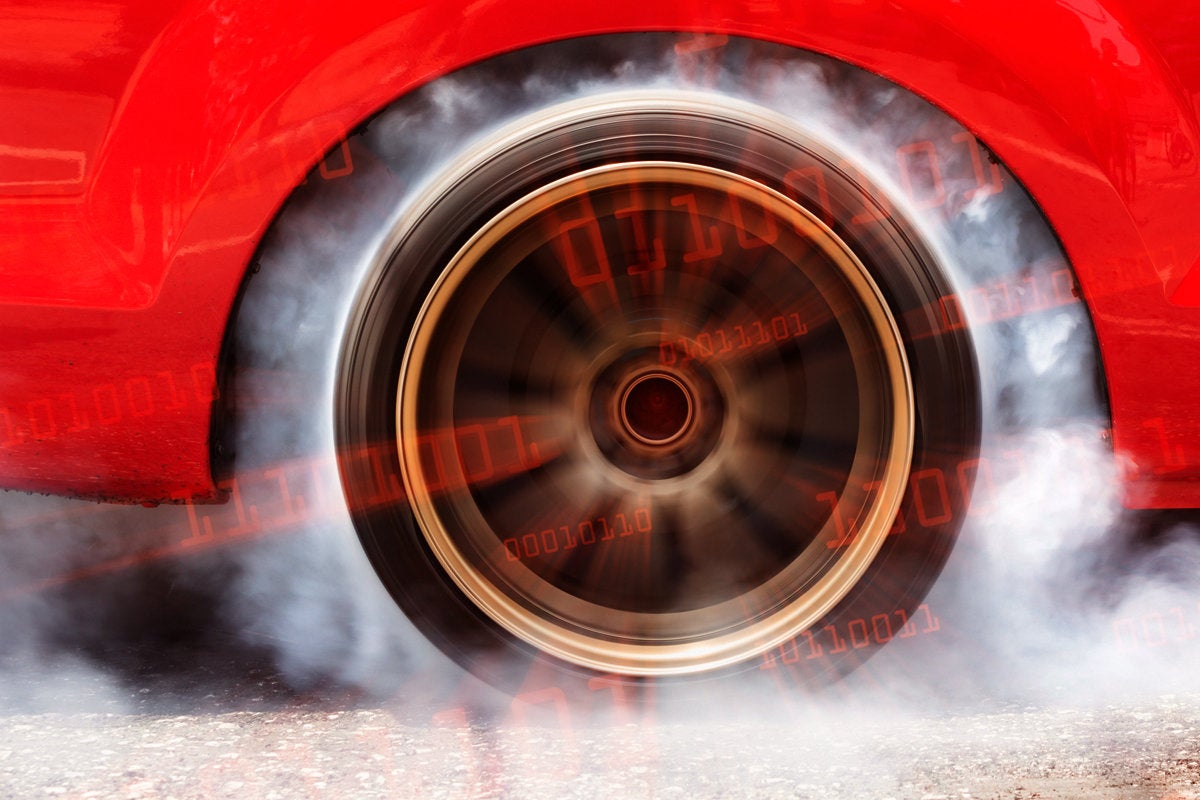
Conclusion
Writing about development is pretty new to me. Therefore, I am eager to hear, if you liked it, if you already know Go and if you are interested in more articles about Go (or other languages).
In case there is enough community demand, I also thought about writing a conclusive tutorial, describing all the Go basics.